Tuyet Nguyen
- Problem Set #1
|
Part 1
|
p1.dbn |
// type program here
// My first program.
// This program makes a simple, vertical
// white line on almost black paper.
paper 85
pen 0
line 20 0 20 100
|
|
Create a program to draw a carefully chosen one line on a paper of your choice.
|
Part 2
|
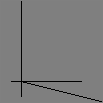 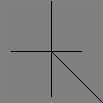 p2.dbn |
// type program here
paper 50
pen 100
set V 80
line 10 V 80 V
line V 5 V 100
line V V 100 0
|
|
Create a program that uses one variable as a means to control a set of 3 lines. Capture 3 instances of the graphic (A,B,C).
|
Part 3
|
p3.dbn |
// type program here
paper 25
pen 85
// creating a filled rectangle
repeat A 0 25
{
line 75 A 100 A
}
|
|
Create a program to draw a single filled rectangle. Again, choose carefully.
|
Part 4
|
p4.dbn |
// type program here
// creates single filled triangle
paper 100
repeat A 15 60
{
pen A
line 15 40 A 85
}
|
|
Create a program to draw a single filled triangle. Use your good judgement.
|
Part 5
|
p5.dbn |
// type program here
paper 5
repeat A 35 65
{
line A A 0 100
}
|
|
Think of all the ways you can draw a filled triangle, and choose one. Utilize the properties of the drawing method you choose, to create a single filled triangle.
|
Part 6
|
p6.dbn |
// type program here
paper 95
// necklace
set A 15
{
set [35 60] A
set [38 45] A
set [50 35] A
set [65 60] A
set [62 45] A
}
|
|
Set 5 dots on a paper of your choice. Evoke an emotion with your choice in placement of dots.
|
Part 7
|
p7.dbn |
// type program here
// draws a simple umbrella
paper 20
repeat A 0 25
{
//code for the top part
set [(A*4) (50+A*4)] 100
set [(100-A*4) (50+A*4)] 100
set [(A*4) (50)] 100
set [(25+A*3) (50+A*6)] 100
set [(100-(25+A*3)) (50+A*6)] 100
set [(50) (50+A*4)] 100
//code for the handle
set [(50) (10+((A*8)/5))] 100
set [(40+((A*4)/10)) (10)] 100
}
|
|
Create a representational picture (i.e. something that looks like something) using just 8 (at maximum) dotted lines.
|
Part 8
|
p8.dbn |
// type program here
paper 0
repeat A 0 100
{
repeat B 0 100
{
set [A B] (A-20)
set [B (A*2)] (A+B)
set [(40+B) (A/2)] B
}
}
|
|
Using a nested loop, fill the entire field with the value of some calculation that can be appreciated. Do not use the Line command.
|
Part 9
|
p9.dbn |
// type program here
paper 100
repeat A 0 15
{
repeat B 0 50
{
repeat C 30 100
{
set [(B+(A*5)) (B*10)] A
set [(10+(B*2))(35+(A+5))] B
set [(B*3) (25+(A*5))] C
}
}
}
|
|
Using a nested loop, create a stippled pattern of dots that can be enjoyed for its complexity. Do not use the Line command.
|
Part 10
|
p10.dbn |
// type program here
paper 0
// top half of lips
{
repeat a 0 50
{
repeat b 50 100
{
pen (a*(3/2))
{
line 25 70 a 50
line 75 70 b 50
}
}
}
}
//bottom half of lips
{
repeat c 0 25
{
repeat d 75 100
{
repeat e 30 50
{
pen (e*2)
{
line 25 30 c 50
line d 50 75 30
line 25 e 75 e
}
}
}
}
}
|
|
Using the Line command, and a (few) nested loop, create an image that evokes a three-dimensional feeling through shading.
|